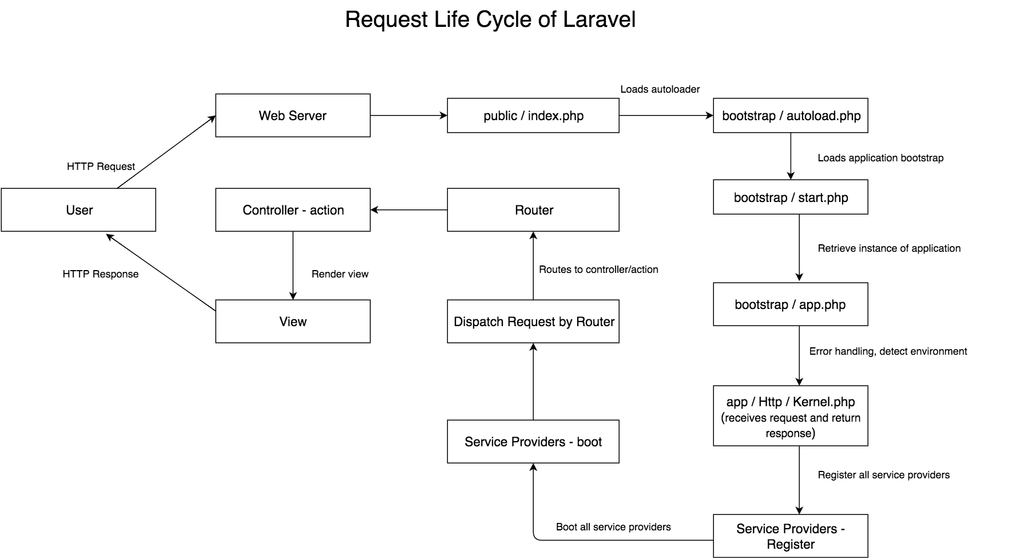
Laravel Request Lifecycle (Expanded)
The Laravel request lifecycle is a fundamental concept that every Laravel developer should understand to build efficient and maintainable web applications. This lifecycle describes the sequence of events that occur from the moment a request enters the application to the point where a response is sent back to the client.
1. Entrance
The request enters the Laravel application through the hire php developer file. This file serves as the entry point and initializes the Laravel framework by requiring the bootstrap files and creating an instance of the Laravel application.
<?php
// public/index.php
// Bootstrap the Laravel application
$app = require_once __DIR__.'/../bootstrap/app.php';
// Run the application
$kernel = $app->make(Illuminate\Contracts\Http\Kernel::class);
$response = $kernel->handle(
$request = Illuminate\Http\Request::capture()
);
$response->send();
$kernel->terminate($request, $response);
?>
2. Kernel Handling
The Illuminate\Foundation\Http\Kernel
class plays a central role in the request lifecycle. The handle
method takes the incoming request and delegates it to the middleware stack for initial processing. The middleware stack is defined in the app/Http/Kernel.php
file and consists of middleware classes that handle various tasks like logging, authentication, and more.
namespace Illuminate\Foundation\Http;
class Kernel extends HttpKernel
{
public function handle($request)
{
$response = $this->sendRequestThroughRouter($request);
// Send the response back to the client
$response->send();
return $response;
}
protected function sendRequestThroughRouter($request)
{
// Handle middleware and route dispatching
}
}
3. Middleware Execution
Middleware are classes that intercept and process HTTP requests and responses. They can modify the request, perform validation, authenticate users, and perform other pre-processing tasks. Middleware are executed in the order they are defined in the Kernel
class, and each middleware can either terminate the request or pass it to the next middleware in the stack.
namespace App\Http\Middleware;
class Authenticate
{
public function handle($request, $next)
{
// Perform authentication logic
return $next($request);
}
}
4. Route Dispatch
Once the request has passed through the middleware stack, Laravel's router takes over. The router matches the incoming request URI to a route definition specified in the routes/web.php
or routes/api.php
file. If a route is matched, the associated controller method or closure is executed to handle the request.
// routes/web.php or routes/api.php
Route::get('/example', 'ExampleController@index');
5. Controller Handling
If a controller is responsible for handling the matched route, Laravel creates an instance of the appropriate controller class. The controller method corresponding to the route is then called, allowing the application to process the request, interact with the database, and prepare a response.
namespace App\Http\Controllers;
class ExampleController extends Controller
{
public function index()
{
// Process the request and prepare a response
return view('example');
}
}
6. Response Creation
After processing the request, the controller returns a response. The response can be a rendered view, a JSON response, a file download, a redirect, or any other type of HTTP response. Laravel provides various response methods and helpers to simplify the creation and customization of responses.
return response()->json(['message' => 'Hello, World!']);
7. Middleware Termination
After the controller method has executed and returned a response, the response is passed back through the middleware stack in reverse order. Each middleware can modify the response headers or content, perform additional logging, or handle any post-processing tasks. Middleware can also short-circuit the response and return a custom response without passing it to subsequent middleware.
namespace App\Http\Middleware;
class LogRequest
{
public function terminate($request, $response)
{
// Log the request and response
}
}
8. Sending the Response
Once all middleware have been processed, and the response has been finalized, Laravel sends the HTTP response back to the client. The server then delivers the response to the requesting client, completing the request lifecycle.
// The HTTP response is sent back to the client
Conclusion
Understanding the Laravel request lifecycle provides developers with insights into how requests are processed, how middleware and routes interact, and how to extend and customize Laravel's behavior using middleware, service providers, and event listeners. This knowledge is essential for building robust, efficient, and maintainable Laravel applications.
Furthermore, Laravel's comprehensive documentation, vibrant community, and rich ecosystem of packages and extensions provide developers with the resources and support they need to explore advanced Laravel features, learn best practices, and stay updated with the latest trends and technologies in web development. By continuously learning and experimenting with Laravel's features and tools, developers can unlock new possibilities, improve their skills, and build innovative and user-friendly applications that resonate with users and deliver tangible business value.
Laravel Request Lifecycle (Further Expanded)
9. Event Dispatching
Laravel provides an event dispatcher that allows developers to subscribe to various events that occur during the request lifecycle. Events provide a way to decouple application logic and make it more maintainable and testable. After the controller method has executed and returned a response, Laravel dispatches any events that were triggered during the request processing, allowing event listeners to perform additional actions or side effects.
// Dispatching an event
Event::dispatch(new RequestProcessed($request));
10. Eloquent ORM
If the controller interacts with a database using Laravel's Eloquent ORM, the ORM allows developers to work with database records as objects, providing a more expressive and intuitive way to query and manipulate data. Eloquent integrates seamlessly with the Laravel request lifecycle, allowing developers to fetch, create, update, and delete records within the controller methods, and return the results as part of the response.
// Fetching records using Eloquent
$users = User::where('status', 'active')->get();
11. View Rendering
Once the controller has processed the request and gathered all necessary data, it typically returns a view as the response. Laravel's Blade templating engine provides a powerful and intuitive way to define and render views. Blade templates can include variables, control structures, and partials, allowing developers to build dynamic and reusable UI components that enhance the user experience.
// Rendering a Blade view
return view('dashboard', ['user' => $user]);
12. Response Sending
After all processing is complete, the response is sent back to the client. Laravel takes care of setting the appropriate HTTP headers and status codes, ensuring that the response is valid and compliant with HTTP standards. The client then receives the response and renders the content, completing the request-response cycle.
// Sending the response back to the client
return response()->json(['message' => 'Request processed successfully'], 200);
13. Termination
After the response has been sent, the terminate
method on the Kernel
class is called, allowing developers to perform any cleanup tasks or additional logging. This method receives the original request and the response that was sent back to the client, providing a final opportunity to inspect or modify the request or response before the application finishes processing the request lifecycle.
namespace Illuminate\Foundation\Http;
class Kernel extends HttpKernel
{
public function terminate($request, $response)
{
// Perform cleanup tasks or additional logging
}
}
Conclusion
Understanding the Laravel request lifecycle provides developers with insights into how requests are processed, how middleware and routes interact, and how to extend and customize Laravel's behavior using middleware, service providers, event listeners, and more. This knowledge is essential for building robust, efficient, and maintainable Laravel applications.
Additional Considerations
It's worth noting that the Laravel request lifecycle can be extended and customized using various Laravel features such as service providers,php development companyevent listeners, and custom middleware. Developers can leverage these features to add additional functionality, implement custom request handling logic, and integrate third-party services to enhance the application's capabilities and provide a richer and more personalized user experience.
Furthermore, Laravel's comprehensive documentation, vibrant community, and rich ecosystem of packages and extensions provide developers with the resources and support they need to explore advanced Laravel features, learn best practices, and stay updated with the latest trends and technologies in web development. By continuously learning and experimenting with Laravel's features and tools, developers can unlock new possibilities, improve their skills, and build innovative and user-friendly applications that resonate with users and deliver tangible business value.